We create custom technologies and deliver solutions. Our work is based on Eclipse and beyond. Want to overcome a challenge? Contact …
Tabris.js 2.2 released
The latest Tabris.js 2.2.0 release brings some interesting new features to the table. A set of new APIs allow to input date and time values, an improved support for properties in TypeScript and several bugfixes make for a great release. Check the rundown below and the official changelog for more details.
New date and time input dialogs
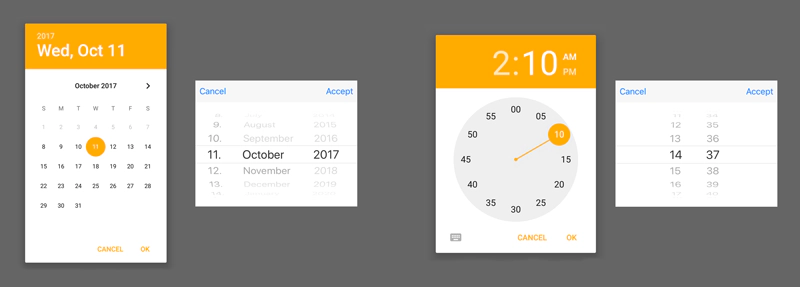
To input date or time with native UI elements, a new [js]DateDialog[/js] and [js]TimeDialog[/js] has been added. These dialogs accept listeners which are informed when a new value is picked.
To open the [js]DateDialog[/js] the following code can be used:
[js] new DateDialog({ date: new Date() }).on({ select: ({date}) => console.log(date), }).open(); [/js]
New “clear button” added to TextInput
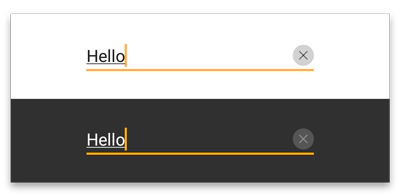
We improved the users experience with a “clear button” on [js]TextInput[/js] with type “search” on Android.
New device vendor property
To retrieve the device’s vendor a new property [js]tabris.device.vendor[/js] has been added. It returns the device manufacturer, e.g., “Apple” or “Samsung”.
Functions as JSX elements
Functions that return a WidgetCollection can now be used as JSX elements. As per convention, their name has to start with an uppercase letter.
The function is called with two arguments:
- the element’s attributes as an object,
- its children (if any) as an array.
An example of this feature would be to call a given widget factory a given number of times:
[js] function Repeat(properties: {times: number}, [callback]: [() => Widget]): WidgetCollection { let result = []; for (let i = 0; i < properties.times; i++) { result[i] = callback(); } return new WidgetCollection(result); } [/js] It can then be used as a regular element: [generic] ui.contentView.append( {() => Hello Again!} ) [/generic]
Note that this example assumes that the element has exactly one child (the [js]callback[/js] function), but the type and number of children are not checked at compile time (attributes are checked). It would therefore be a good idea to check the type of the children at runtime.
Improved support for custom properties in TypeScript
When creating a custom subclass of an existing Tabris widget, new properties don’t behave the same as the built-in ones out of the box. Getting the constructor, as well as the [js]set()[/js] and [js]get[/js] methods to accept the new properties, have previously been rather tedious. Now, with the with the addition of the tsProperties property and the [js]Properties[/js] and [js]Partial[/js] interfaces, this process has been greatly simplified.
The following example shows a custom UI component with the full support of its new properties:
[js] import {Composite, Partial, Properties} from ’tabris';
class MyCustomForm extends Composite {
public tsProperties: Properties & Partial<this, ‘foo’ | ‘bar’>
;
// initializing plain properties is a must for “super” and “set” to work as a expected. public foo: string = null; public bar: number = null;
constructor(properties?: Properties) { super(properties); }
} [/js]
For more detailed information please read the section “Interfaces” in the Tabris.js documentation.
How to get started with Tabris.js 2.2
- Install the new Tabris.js Developer App on your device.
- Try out the examples bundled with this app.
- Run your code snippets from the playground, our online Tabris.js editor.
To start developing real apps,
- Install the latest Tabris CLI on your machine: [shell]npm install -g tabris-cli[/shell]
- Type [shell]tabris init[/shell] in an empty directory – this will create a simple example app.
- Type [shell]tabris serve[/shell] and load it in the Developer App.
The documentation contains everything you need to know (tip: try the doc search). Beginners can find a step-by-step guide in this ebook. If you have questions or comments, you’re also invited to join the community chat.
Happy coding!